Create an Interactive Harry Potter Marauder’s Map with a Raspberry Pi-Powered Display
Welcome to Engineering Evening, where we’re about to embark on a magical journey inspired by the world of Harry Potter! In this guide, we’ll explore the creation of a Marauder’s Map displayed on a 32″ television enclosed in a custom-built wood display case. Get ready to infuse your living space with a touch of wizardry and charm. Let’s dive into the details and weave some digital magic using a Raspberry Pi.
Requirements:
- Raspberry Pi 4 (Model B)
- Micro SD Card with Raspberry Pi OS installed (16GB+ recommended)
- Power supply for Raspberry Pi
- 32″ Television
- Custom-built wood display case
- RFID-RC522 sensor module
- GPIO break out board (optional, but is really helpful)
- Breadboard (optional, for easy connections)
- HDMI cable
- USB keyboard & mouse for initial setup
- RFID cards or tags
- Harry Potter wand/3d printed wands/make your own wands
- Access to a web browser
- Video of the Marauder’s Map
Note: We support our efforts bringing you these free tutorials through affiliate links. These links are affiliate links which means we do get paid if you click on them and make a purchase. These are the exact products we ordered and used, so you and rest assured there’s no funny business of linking to junk products.
If you appreciate our content, please support us by using our links.
Step 1: Setting Up VLC Player on Raspberry Pi 4 Before we delve into the enchantment of the Marauder’s Map, let’s ensure your Raspberry Pi 4 is ready to perform its magic. Follow the guide on our site that explains how to set up VLC to be controlled via HTTP requests using the python-vlc-http library.
Step 2: Creating the Marauder’s Map Video Loop Refer to our guide on how to set up a Raspberry Pi to loop a video in VLC upon booting. Follow that tutorial, but instead of launching VLC player directly, you will change the content of the autovlc.desktop
file (feel free to change the name of that file) to launch your Python script instead. The Python script will then launch VLC for you. This is how you would modify that file to launch VLC instead:
[Desktop Entry]
Encoding=UTF-8
Type=Application
Terminal=false
Exec=python [python file location]
Be sure to replace [python file location]
with the actual location of your file.
Step 2.1: Choose or create a mesmerizing loopable video that captures the essence of the Marauder’s Map. The Loopable Harry Potter Marauders Map that we created is available for purchase on our online store. The video doesn’t need to be long, just long enough to impress your guests, but not so long you lose their attention. The video we produced is only about 30 seconds long, which worked out very well.
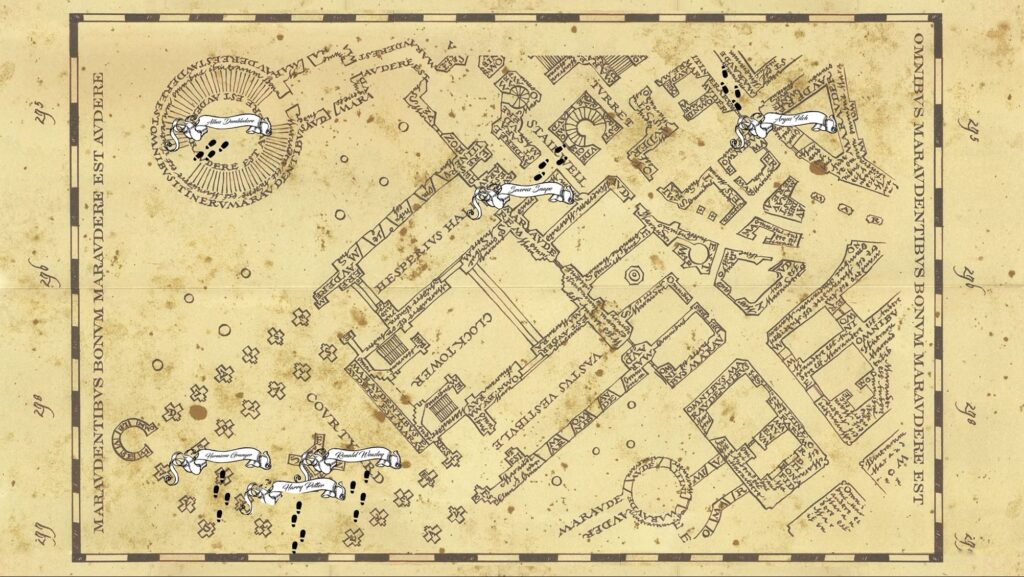
Step 3: Integrating RFID Tag Control for Extra Wizardry Enhance your Marauder’s Map experience by incorporating RFID technology. Follow our guide on using a Raspberry Pi to read an RFID tag and then use it to trigger commands to VLC Player. You can program RFID cards or tags to trigger specific VLC commands, such as starting, pausing, or changing the video loop. For this project, we set up the code to play one loop of the video each time any RFID tag was detected.
We found these RFID stickers were small and worked great to attach to a “wand”.
Step 4: Assembling the Components
- Place the Raspberry Pi inside the custom-built wood display case, ensuring proper ventilation.
- Connect the HDMI cable from the Raspberry Pi to the television.
- Power up the Raspberry Pi and the television using suitable power supplies.
- Attach the RFID-RC522 sensor to the Raspberry Pi using jumper wires and mount the sensor on the outside of your display case where it can easily be accessed.
- Tape or glue the RFID tags to the wands you will be using to activate the Marauder’s Map.
Step 5: Sample Python Code for RFID-Triggered Commands Below is the Python script that we used to control VLC Player based on RFID triggers (including the debug lines that print what’s happening to the terminal):
from mfrc522 import MFRC522 #RFID tag reader
import time #So we can introduce delays
from python_vlc_http import HttpVLC #VLC Player Controls
from subprocess import Popen
#Launch VLC and load [media file]
Popen(['vlc','[media_file]'])
time.sleep(3) #Wait 3 seconds for VLC to open
reader = MFRC522()
# Function to read the blocks on the RFID tag
def read(trailer_block, key, block_addrs):
(status, TagType) = reader.MFRC522_Request(reader.PICC_REQIDL)
if status != reader.MI_OK:
return None, None
(status, uid) = reader.MFRC522_Anticoll()
if status != reader.MI_OK:
return None, None
id = uid
reader.MFRC522_SelectTag(uid)
data = []
text_read = ''
if status == reader.MI_OK:
for block_num in block_addrs:
block = reader.MFRC522_Read(block_num)
if block:
data += block
if data:
text_read = ''.join(chr(i) for i in data)
reader.MFRC522_StopCrypto1()
return id, text_read
trailer_block = 11
key = [0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF]
block_addrs = [1,2,3,4,5,6,7,8,9,10]
id = ""
vlc_client = HttpVLC('http://[VLC IP Address]:[VLC Port]', '', '[VLC Password]')
time.sleep(3)
print(vlc_client.state())
if vlc_client.state() == "playing":
vlc_client.pause()
vlc_client.seek(3)
while 1: #Loop indefinitely
print("reading...")
id, text = read(trailer_block, key, block_addrs)
while (str(id) == "None"): #Loop until we find an RFID Tag
id, text = read(trailer_block, key, block_addrs)
block10 = reader.MFRC522_Read(10) #Read Block 10, this is where we would store our data
print("Block 10: " + str(block10))
try:
print("Block 10.0: " + str(block10[0]))
#Do additional fun stuff here!
vlc_client.seek(3) #Seek the video to the 3 second mark
vlc_client.play()
print('VLC Playing')
time.sleep(28) #Wait 28 seconds (let the video play for 28 seconds)
vlc_client.pause()
print('VLC Paused')
except:
print("Incomplete read")
time.sleep(1)
Replace [media_file]
, [VLC IP Address]
, [VLC Post]
and [VLC Password]
with the actual file and VLC settings of your VLC server. Hint: If VLC is running on the same device as this Python script, the IP Address is 127.0.0.1 and the default Port is 8080
The print
lines of code are only there to help you see where in the script it is for testing. Those lines of code can safely be removed or commented out by adding a # in front of the print
statement.
Important Note: These RFID tags are more complex than some others and they store an array of information. Some RFID tags only store a single value (usually text or a number), so you may need to adapt the code depending on what kind of RFID tag you are using. For these RFID tags, through experimentation we discovered that we can only write to the first 4 items of a sector (0-3), which is more than enough for our needs.
Upgrade Idea: RFID tags can be programmed with unique values. That means you could store information on the RFID tags (not all RFID tags allow you to write information to them). You could add additional parts to your code that counted how many times each wand is used. That’s what we did and here’s the code we used (insert this code just after the #Do additional fun stuff here!
line in the code above):
#Update the tag to track the number of times it has been detected
block10[0] = block10[0] + 1 #increment the counter in block 10.0
try:
reader.MFRC522_Write(10, block10)
except:
print("Error writing!")
#Check to make sure the counter was updated
try:
block10 = reader.MFRC522_Read(10)
print("Block 10: " + str(block10))
except:
print("Couldn't read")
Epic Upgrade Idea: Since each RFID tag can have unique values, you could update this program to play a different portion of the video (seek to a different place before playing). For instance, we plan to have 2 versions of the map: If a Death Eater’s wand is used, it will show other Death Eaters on the map, but if another wand is used, it will show Hogwarts students on the map!
Step 6: Create Your “Magic Wands” Now it’s time to create your magic wands. With the RFID tag stickers we used and recommend, you can make pretty much anything into a magic wand. For our party, we 3D printed wands, but you could also use a pencil, chopsticks or even a banana! All you need to do is stick one of your RFID tags onto the “wand”.
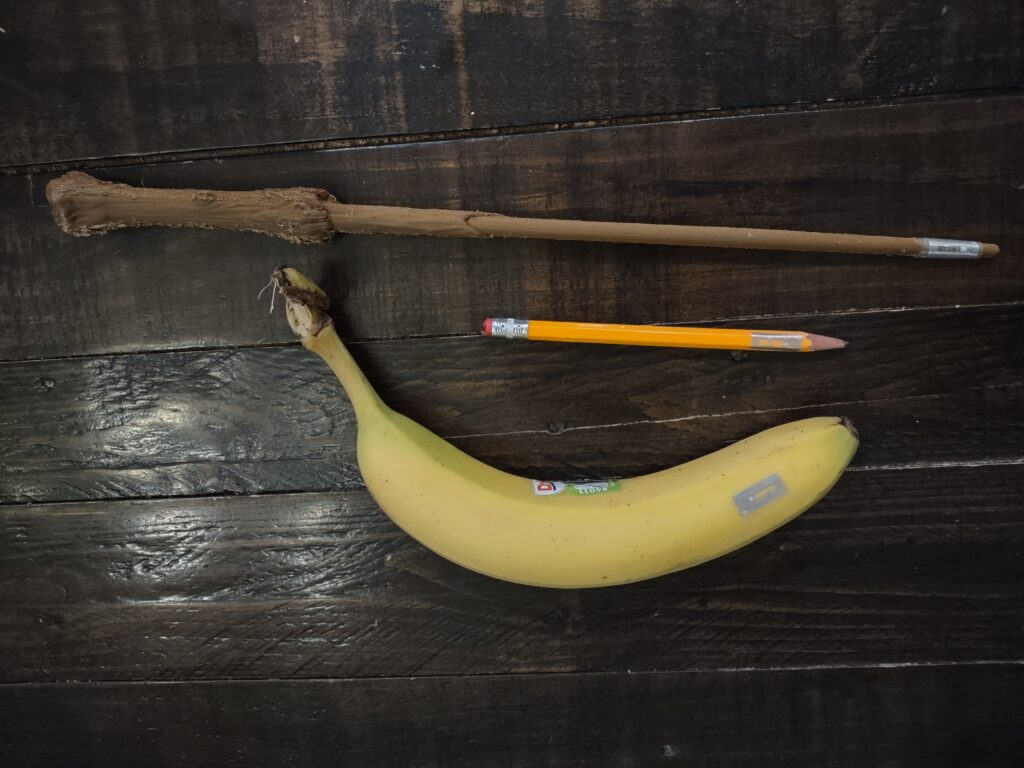
Step 7: Revel in the Magic Power up your Raspberry Pi then run your Python script and watch as the Marauder’s Map comes to life! You can experiment with the RFID tags to control VLC Player. The enchanted map will respond to your magical commands.
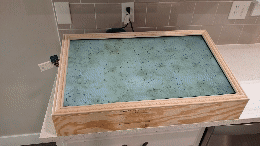
Conclusion: Embracing the Magic of Technology Congratulations! You’ve successfully combined the wizarding world of Harry Potter with the technological wonders of Raspberry Pi and VLC Player. Your Marauder’s Map display is sure to captivate anyone who enters your space. Feel free to experiment further, adding more RFID triggers or exploring additional VLC features. May your days be filled with the magic of technology and the enchantment of Hogwarts!